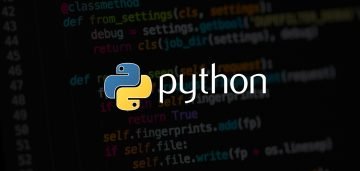
Python is a programming language that is visually similar to Ruby, has a diverse community with numerous ties to Linux and academia, works with the Django framework, and is used by Google, Youtube, Dropbox, and more. Because of their similar nature, Python should be of interest to Ruby developers.
Python takes a more direct approach to programming, with a primary goal of making everything visible to the programmer. Although this might sacrifice a bit of elegance found in Ruby, it gives Python an advantage when it comes to learning how to use and debug the language.
Since many developers prefer to work within an IDE (Integrated Development Environment), it only stands to reason that there’s such a tool specific to Python. That tool is IDLE (Integrated DeveLopment Environment). IDLE is an IDE for Python, written in Python, and based on Tkinter (with bindings to the TK widget set).
Where IDLE really shines is with those just starting out with Python. IDLE includes a fairly basic feature set:
Multi-window editor with auto-complete, smart indent, and more.
Python shell with syntax highlighting.
Integrated debugger which includes stepping, persistent breakpoints, and call stack visibility.
IDLE is available for Linux, so let’s see how to install and use this IDE on Ubuntu Linux 19.10.
Installation
Fortunately, IDLE is found in the Linux standard repositories, so the installation is actually quite simple. Here are the steps for a successful installation:
Open a terminal window.
Update apt with the command sudo apt-get update.
Install IDLE with the command sudo apt-get install idle3 -y (Figure 1).
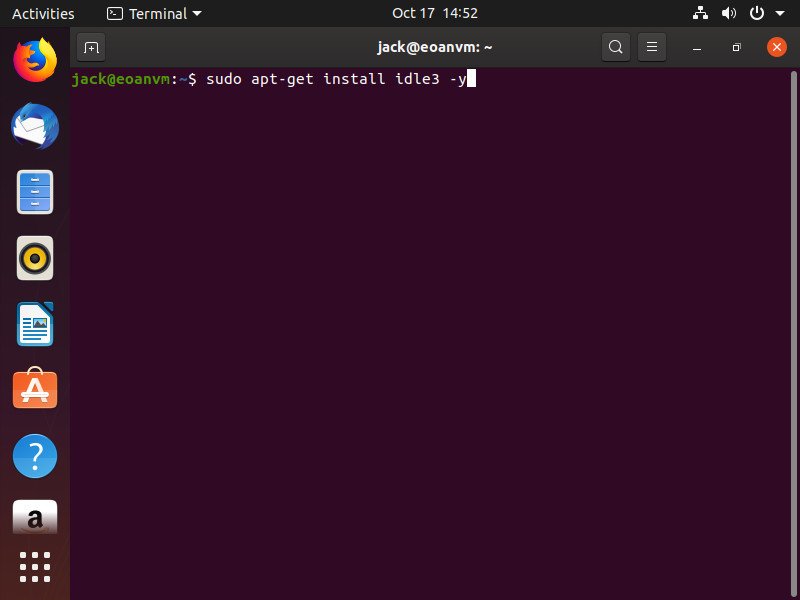
Once the installation completes, you should be able to launch the application from your desktop menu (Figure 2).
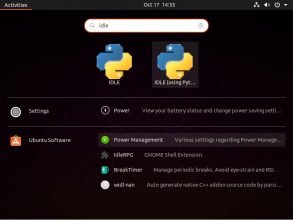
Creating Your First Program
Let’s create a very simple Python program with IDLE. We’ll use the ever-popular “Hello, World!” example.
From the Idle prompt (Figure 3), type:
print(“Hello, World!”)
Hit Enter on your keyboard and you’ll see Hello, World! Displayed.
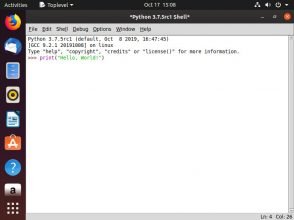
Now let’s use a less basic example: A sample Python program that serves as a simple calculator. The code for this application looks like this:
# Program make a simple calculator that can add, subtract, multiply and divide using functions
# This function adds two numbers
def add(x, y):
return x + y
# This function subtracts two numbers
def subtract(x, y):
return x – y
# This function multiplies two numbers
def multiply(x, y):
return x * y
# This function divides two numbers
def divide(x, y):
return x / y
print(“Select operation.”)
print(“1.Add”)
print(“2.Subtract”)
print(“3.Multiply”)
print(“4.Divide”)
# Take input from the user
choice = input(“Enter choice(1/2/3/4):”)
num1 = int(input(“Enter first number: “))
num2 = int(input(“Enter second number: “))
if choice == ‘1’:
print(num1,”+”,num2,”=”, add(num1,num2))
elif choice == ‘2’:
print(num1,”-“,num2,”=”, subtract(num1,num2))
elif choice == ‘3’:
print(num1,”*”,num2,”=”, multiply(num1,num2))
elif choice == ‘4’:
print(num1,”/”,num2,”=”, divide(num1,num2))
else:
print(“Invalid input”)
From within IDLE, click File > New File. In the resulting window, paste the above code (Figure 4), making sure to keep the same indention you see above.
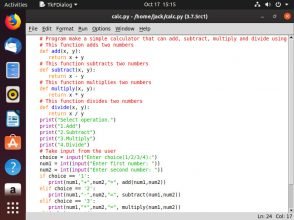
Click File > Save (so you have a copy of the file on hand) and name the file calc.py. Now click Run > Run Module. You will see the program runs and asks the user to select an option (Figure 5).
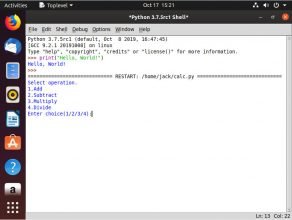
Congratulations, you’ve run your first full Python program in IDLE.
Using the Debugger
A debugger is a way to check for problems in your code. The IDLE debugger is easy to use, once you know how. Here are the steps:
1. Start IDLE.
2. Open the calc.py program you saved from earlier.
3. Back in the main window, click Debug > Debugger.
4. You should now see the IDLE debugger is on (Figure 6).
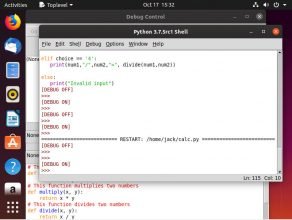
5. Go back to the window displaying the code and click Run > Run Module.
6. Go back to the Debugger window and click the Go button (Figure 7).
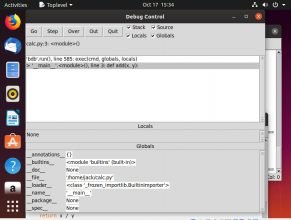
7. Back in the main window, you should see the program execute, waiting for input. As you step through the program, if there are any problems with the code, the Debugger will help you find them.
And that’s the gist of how to use the IDLE Python Integrated Development Environment. By getting up to speed with this tool, you might find it easier to work with third-parties, such as ruby development outsourcing, python development outsourcing, or working with ruby development projects.
Even if you aren’t a Ruby or Python developer, if you’re interested in learning how to code, working with IDLE is a great place to start.
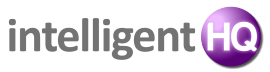
Founder Dinis Guarda
IntelligentHQ Your New Business Network.
IntelligentHQ is a Business network and an expert source for finance, capital markets and intelligence for thousands of global business professionals, startups, and companies.
We exist at the point of intersection between technology, social media, finance and innovation.
IntelligentHQ leverages innovation and scale of social digital technology, analytics, news, and distribution to create an unparalleled, full digital medium and social business networks spectrum.
IntelligentHQ is working hard, to become a trusted, and indispensable source of business news and analytics, within financial services and its associated supply chains and ecosystems